tl;dr: New mini-book series about Node-based code and networking patterns here: http://nodepatternsbooks.com
I first started playing around with Node back in 2009, shortly after version 0.1 was released. A lot of things have changed insince then: some API changes, NPM happened and grew, the community grew and matured, the software industry adopted it. But what brought me to Node initially is still there: the low level API that allows you to easily create networked programs in JavaScript; the module system and NPM make it convinient to embrace the UNIX philosophy: it’s easy to create and compose short, simple modules and services that do one thing right.
Node has grown a lot since then: there is now a myriad of NPM packages to choose from, new frameworks almost every week. Not only does the Node and NPM ecosystem enables you to easily assemble and create a traditional HTTP Server, it also makes it convenient for you to create and invent new network services that use JavaScript to build Peer-to-Peer, Distributed, Real-time communications and many other types of complex systems.
Unlike opinionated frameworks in other languages, Node and most of Node frameworks don’t impose any back-end database, network protocols or even directory structure. It’s up to the developer to choose what they think better fits the project and the development team.
For more than six months I’ve been developing this new series of short books aiming to extract and present some of the common patterns I have used and seen used throughout several types of applications. Today I’m releasing the first batch, covering some small-scale code and large-scale networking patterns, aiming to build up a portfolio of concepts, libraries and code that I hope you find interesting and useful. Here they are:
Modules

The first short book of this series is a short introduction that sets the foundation of the next books to come. It shows what you can do with JavaScript and the Node module system to create and consume different types of modules: Singletons, Closure-based and prototype-based pseudo-classes, façades and others.
Flow Control

In JavaScript, any operation involving the network or the file system is always asynchronous. Once you start implementing complex logic, coordinating I/O operations can become a challenge in Node. This book will give you basic patterns and tools that will allow you to master and control asynchronous flow in Node. It covers the standard Callback pattern, queues, Event Emitters and Streams.
Work Queues
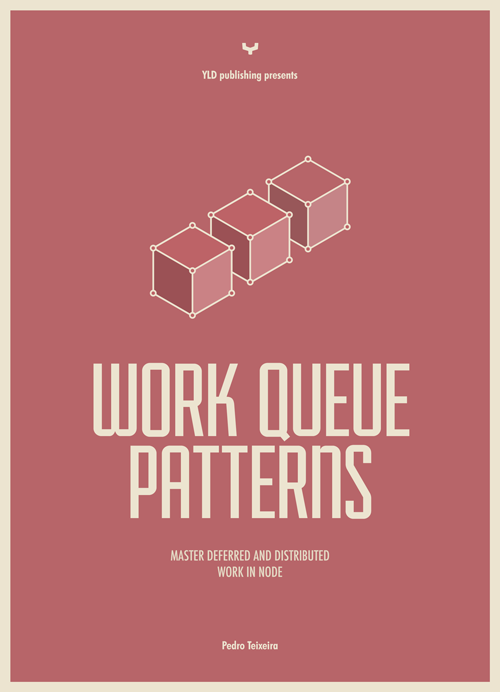
Most applications have some type of work that can be performed independently from the client request cycle. You can defer these types of work into a memory queue or, to survive crashes, a persisted queue. A distributed queue separates the work producer from the work consumer, allowing you to perform work on a different process.
This book will show you how to create and consume work in a local or distributed environment using Node.
Configuration Patterns

Any application normally needs some environment-specific configuration details: the hostname and port of the database server, the access token for the mail delivery service API and many others. Once your application starts spanning more than one machine, the distribution of the configuration can start becoming troublesome.
This book shows some approaches for managing and distributing the configuration of your Node application.
Networking Patterns
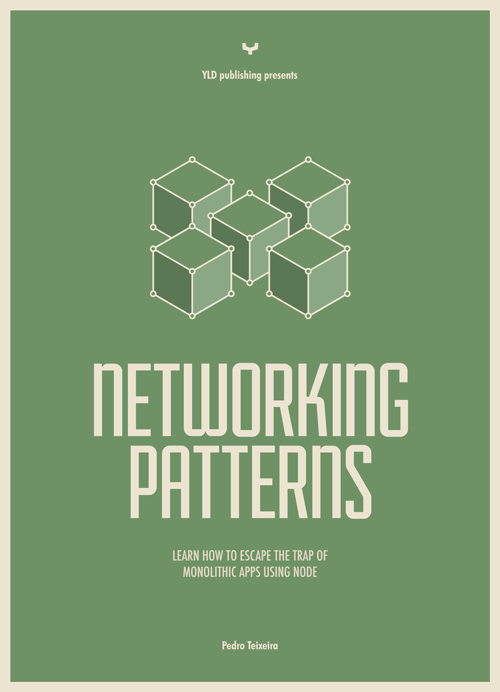
Building an application using a web framework can soon lead to one big chunk of monolithic code. This happens not because of the web framework itself, but mostly because building distributed services can be a hard task. Fortunately, Node makes it easy to build networked services, allowing you to spread your application logic into a set of processes that communicate with each other.
This book shows you how to build and consume TCP-based services like streaming services, multiplexing streams, remote emitters, RPC streams and other types of network-based servers and clients.
Databases — Volume I

Node.js has been designed to do quick and efficient network I/O. It’s event-driven streams make it ideal to be used as a kind of smart proxy, often working as the glue between back-end systems and clients. Node was originally designed with that intention in mind, but meanwhile it also has been successfully used to build traditional web applications: an HTTP server that serves HTML pages or replies JSON messages and uses a database to store the data. Even though web frameworks in other platforms and languages have preferred to stick with traditional open-source relational databases like MySQL or PostgreSQL, most of the existing Node web frameworks (like Express, Hapi and others) don’t impose any database or even any type of database at all. This bring-your-own-database approach has been in part fed by the explosion in the variety of database servers now available, but also by the ease with which the Node module system and NPM allow you to install and use third-party libraries.
In this book we will analyze some of the existing solutions for interacting with some types of databases and what interesting uses can you give them. This first short book on databases starts with some of my favourites: LevelDB, Redis and CouchDB.